PayUBolt SDK Integration
PayUBolt SDK will provide a way to integrate in-app UPI payment in merchant app with their own UI. They can manage the checkout options on their checkout screen. Features included in this SDK are:
- Registration - This SDK provides APIs for device binding and registration. Merchant App can consume these APIs and build their own registration journey.
- Payment - This SDK provides payment and verify transaction APIs. Merchant App can consume these APIs to build payment journey as well.
- Management - This SDK provides account management APIs. Merchant App can check balance, set/change pin and add/delete account in their own user journeys.
This integration involves the following steps:
Later, you can integrate the following flows:
For hash generation logic and Listener/Callback integration, the Hash generation logic and o Listener or Callback logic sub-sections.
Prerequisites
- Minimum Android SDK Version - 23 and above.
- Compile SDK Version - 31 and above.
- The following .aar (Android archive) files provided by PayU during onboarding:
- NPCI Secure Component
- AXIS Olive
Step 1: Add permissions to Manifest file
Update the manifest file to include the following so that permissions are provided for SDK:
// To send SMS for device binding
<uses-permission android:name="android.permission.SEND_SMS"/>
// To check current network state
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
// To access internet for API calls
<uses-permission android:name="android.permission.INTERNET" />
// To get sim details from devices below or equal to 29
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
// Too get sim details from devices above 29
<uses-permission android:name="android.permission.READ_PHONE_NUMBERS" />
// To provide location details for transaction
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
Step 2: Include Bolt SDK and AAR Files
To include the PayU UPI Bolt SDK in your project, add the following code snippet to your app’s build.gradle
.
implementation 'in.payu:payu-upi-bolt-axis-wrapper-sdk:1.0.0' // PayU AXIS Wrapper
implementation 'in.payu:payu-upi-bolt-sdk:1.0.0' // PayU Bolt SDK
Add the .aar files provided by PayU during onboarding. in the libs directory of your android module and add these in module level build.gradle. For the list of files, refer to Prerequisites.
api(files("$projectDir/libs/SecureComponent-release-prod_05062024_9d3904ab.aar")) // NPCI .aar
api(files("$projectDir/libs/oliveupi-payu-release_PROD_02-12-2024_2.0.2.aar")) // AXIS .aar
The screenshot of libs directory is similar to the following:
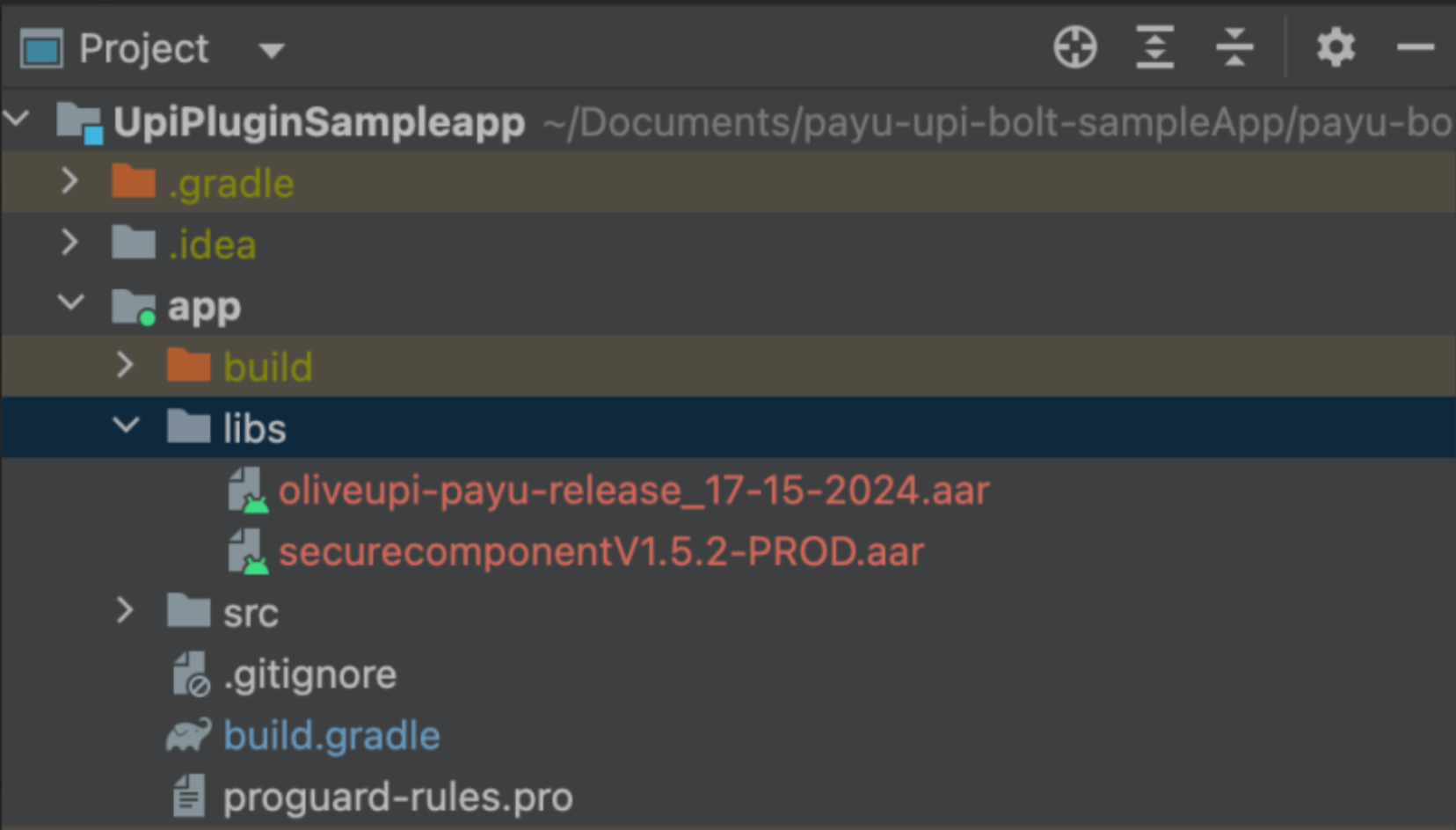
Step 3: Initialize the SDK
It is used to initialize the SDK. This method returns a object that will be used to access other methods available in PayUUPIBoltUI
.
val bolt = PayUUPIBolt.getInstance(
activity: AppCompatActivity,
config: PayUUPIBoltConfig,
hashGenerationListener: PayUHashGenerationListener
)
The following fields are needed as a request for this API:
Parameter | Definition |
---|---|
activitymandatory | AppCompatActivity Calling activity of the merchant App |
configmandatory | PayUUPIBoltUIConfig Config includes the below fields. |
hashGenerationListenermandatory | PayUHashGenerationListener Callback listener for hash generation |
The PayUUPIBoltConfig includes the following fields:
Fields | Definition |
---|---|
merchantKeymandatory | String PayU Merchant Key |
phonemandatory | String Phone number for registration |
emailmandatory | String Customer Email Id |
pluginTypemandatory | String Array List of Supported Banks (“AXIS, HDFC”) |
isProdoptional | Boolean Prod - ture, staging - false |
excludedBanksIINsoptional | String Array List of Bank’s IIN to exclude |
requestIdmandatory | String Unique reference ID |
Response
Response Params | Definition |
---|---|
PayUUPIBolt | PayUUPIBolt object for invoking SDK APIs |
Callback:
After the SDK is initialised, use the same object to call the sdk methods.
De-initialise PayUBolt SDK
reset
: This method is used to deinitailise the bolt object.
`PayUUPIBolt.reset()`
Listener or Callback logic
Listerner/Callback contains 3 methods where the merchant app will get the API response and hash-related callbacks
S.No. | Listener | Description |
---|---|---|
1 | fun generateHash(map: HashMap<String, String>, hashGenerationListener: PayUHashGeneratedListener) | For hash generation, refer to Hash generation logic sub-section |
2 | fun onPayUSuccess(response: PayUUPIBoltResponse) | It will contain success response. |
3 | fun onPayUFailure(response: PayUUPIBoltResponse) | It will contain failure response. |
PayUUPIResponse
Fields | Data Type | Definition |
---|---|---|
responseType | Integer | Refer to Response type. |
code | Integer | Error or success code. Refer to Response codes . |
message | String | Error or success message. Refer to Response codes . |
result | Object | Response data |
Note: If result object is null or empty. Kindly use the response from message
Response type
Response Type | Response Code | Definition |
---|---|---|
REQUEST_UPI_BOLT | 100 | UPI Bolt Status |
REQUEST_TRANSACTION | 124 | Register And Pay |
REQUEST_MANAGE | 125 | UPI Management |
Response codes
Codes | Message |
---|---|
0 | Success |
1 | Fail/ Invalid Response/ Missing params |
2 | User canceled the transaction |
100 | Transaction timeout |
101 | Hash missing |
102 | Something went wrong |
103 | Handshake failed |
104 | UPI bolt not supported |
105 | Device not supported for UPI Bolt |
106 | Permission missing |
107 | Sim info not available |
108 | Device binding failed |
109 | Initiate pay failed |
110 | Dispute already exists |
501 | No internet connection |
Hash Generation logic
The PayU SDKs use hashes to ensure the security of the transaction and prevent any unauthorized intrusion or modification.
For generating and passing dynamic hashes, the merchant will receive a call from the generateHash()
method of PayUUPIBoltUiListener
. The generateHash()
method is called by the SDK each time it needs an individual hash.
fun generateHash(map: HashMap<String, String>, hashGenerationListener: PayUHashGeneratedListener): Merchant will get map with type of hash and hash string as value of map.
They have to sign that string using salt to create hash value and pass that to hashGenerationListener.onHashGenerated().
In the map you have to check for three keys to generate hash.
1. hashString
2. hashName
3. postSalt
At the end of that hashString append your salt and use SHA-512 algo on that final string to generate hash.
Note: If you got postSalt also in the map, first use hash string append salt and then append postSalt value to that string and use SHA-512 algo on that final string to generate hash.
Once the hash is generated use hashGenerationListener parameter to pass the hash to SDK. Example code:
val hashMap: HashMap<String, String> = HashMap()
hashMap[hashName] = hash //hashName is the value you got in map and hash is the hash value.
hashGenerationListener.onHashGenerated(hashMap)
Integrate Registration Flow
The following methods are used to integrate registration flow:
- Check if UPI Bolt is enabled
- Get registered mobile number
- Get subscriber info
- Check device status
- Initiate SDK
- Fetch bank list
- Fetch accounts of a selected bank
- Set VPA
- Set PIN
Check if UPI Bolt is enabled
Use the isUpiBoltEnabled
method to check whether the UPI bolt is enabled for the merchant or not enabled.
bolt.core.isUpiBoltEnabled(callback: PayUUPIBoltCallBack)
The following parameters are needed as a request for this API:
Paramater | Definition |
---|---|
callback mandatory | PayUUPIBoltCallback Ref. Listener/Callback logic section. |
Response: Response type : REQUEST_UPI_BOLT. For more information, refer to Response type.
Get registered mobile number
Use the getRegisteredMobile
method to get already registered mobile number.
bolt.core.getRegisteredMobile(): String
The following parameters are needed as a request for this API:
Paramater | Definition |
---|---|
Mobile | Registered Mobile Number |
Get subscriber info
Use the getSubscriberInfo
method to get SIM info from device.
bolt.core.getSubscriberInfo(mobile: String, callback: PayUUPIBoltCallBack)
Callback reference:
For callback logic refer to Listener or Callback logic sub-section.
The following parameters are needed as a request for this API:
Parameter | Definition |
---|---|
mobilemandatory | String Mobile number to be used for registration. |
callbackmandatory | PayUUPIBoltUICallBack This parameter contains the callback. For callback logic refer to Listener or Callback logic sub-section. |
The response is PayUSimInfo
array list that contains the following fields:
Field | Definition |
---|---|
mobileNumber | String Mobile number |
slotIndex | String SIM slot index |
subscriberId | String SIM subscription id |
carrierName | String Name of carrier |
Check device status
Use the checkDeviceStatus method to check the device binding status.
bolt.core.checkDeviceStatus(mobile: String, subscriptionId: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
mobilemandatory | String Mobile number to be used for registration |
subscriptionIdmandatory | String SubscriptionId of Mobile Number |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is CHECK_DEVICE_STATUS.
Initiate SDK
Use the initiateSDK
method to start device binding and triggering SMS.
bolt.core.initiateSDK(subscriptionId: String, phone: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
subscriptionId | String SubscriptionId of Mobile Number |
mobile | String Mobile number to be used for registration |
callback | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_SDK_HANDSHAKE
Fetch bank list
Use the fetchBankList
method to fetch a list of all banks.
bolt.core.fetchBankList(callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_LIST_BANKS
The response is PayUBankData
array list that contains the following fields:
Field | Definition |
---|---|
name | String Name of bank |
iin | String Issuer identification number of bank |
ifsc | String IFSC code |
logo | String Bank logo url |
bankCode | String Unique identification code for the bank |
Fetch accounts of a selected bank
Use the fetchAccountsWithIin
method to fetch accounts of selected bank.
bolt.core.fetchAccountsWithIin(iin: String, bankName: String, bankCode: String?, vpa: String?, requestType: String?, isCCTxnEnabled: Boolean, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Field | Definition |
---|---|
iin mandatory | String Issuer identification number of bank |
bankname mandatory | String Name of bank |
bankCodeoptional | String Unique identification code for the bank |
vpa optional | String UPI handle |
requestType optional | String Only applicable for HDFC and contain any of the following:- A: Add account - R : New registration |
isCCTxnEnabled mandatory | String The default value is false. Set it true if bank is CC. |
callback mandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_FETCH_ACCOUNT_V3
The response is PayUCustomerBankAccounts
array list that contains the following fields:
Field | Definition |
---|---|
bankName | String Name of bank |
bankCode | String Unique identification code for the bank |
bankAccounts | Refer to PayU Account Detail Parameters |
Set VPA
Use the setVpa
method to update the vpa of the registered account.
bolt.core.setVpa(accountDetail: PayUAccountDetail, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail Parameters |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section |
Response: Response type is REQUEST_SAVE_VPA_V3
Set PIN
Use the setPin
method to set PIN for new account..
bolt.core.setPin(accountDetail: PayUAccountDetail, cardNo: String, exp: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail section |
cardNomandatory | String Last 6 digit of user’s card number |
expmandatory | String Month and Expiry of user’s card number (format: MM/YYYY) |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_ACCOUNT_MOBILE_REG
Integrate Repeat Flow
Fetch linked accounts
Use the fetchLinkedAccounts
method to fetch registered account with the device.
bolt.core.fetchLinkedAccounts(callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_ALL_ACCOUNTS_V3
The response is PayUCustomerBankAccounts
array list that contains the following fields:
Field | Definition |
---|---|
bankName | String Name of bank |
bankCode | String Unique identification code for the bank |
bankAccounts | PayUAccountDetail Refer to PayU Account Detail Parameters |
Integrate Payment Flow
The following methods can be used to integrate payment flow:
Initiate payment
Use the pay
method to initiate payment..
bolt.core.pay(paymentParams: PayUUPIBoltPaymentParams, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
paymentParamsmandatory | PayUAccountDetail Refer to |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
paymentParams field description
Parameter | Description | Example |
---|---|---|
amountmandatory | String Total transaction amount. | 100.0 |
txnIdmandatory | String It should be unique for each transaction.Cannot be null or empty and should be unique for each transaction. The maximum allowed length is 25 characters. It cannot contain special characters like: - "_,$,%,&, etc" | 4567890 |
productInfomandatory | String Information about the product. | "ProductInfo" |
firstNamemandatory | String Customer’s first name. | "Firstname" |
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail Parameters | |
udf1 optional | String User defined field | |
udf2 optional | String User defined field | |
udf3 optional | String User defined field | |
udf4 optional | String User defined field | |
udf5 optional | Numeric User defined field |
Response: Response type is REQUEST_PAY
Check transaction status
Use the checkTransactionStatus
method to check the transaction status.
bolt.core.checkTransactionStatus(txnId: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
txnIdmandatory | String Transaction ID used for payment |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_CHECK_PAYMENT_STATUS
Field | Definition |
---|---|
result | JSON JSON key “status” contains transaction status value. |
Cancel transaction
Use the cancelTransaction
method to cancel the current transaction.
bolt.core.cancelTransaction(callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_CANCEL_TRANSACTION
Integrate Management flow
The following methods can be used to integrate management flow:
- Fetch transaction history
- Check balance
- Remove account
- Change mPIN
- Fetch VPA profile
- Save VPA
- Delete VPA
- Raise query or dispute
- Fetch query list
- Deregister
- Check required permissions
- Check clear cache
- Clear data
Fetch transaction history
Use the fetchTransactionHistory
method to fetch the transaction history.
bolt.core.fetchTransactionHistory(fromDate: String, toDate: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
fromDatemandatory | Transaction start date from which transaction history is required |
toDatemandatory | Transaction end date until which transaction history is required |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_TRANSACTION_HISTORY_V3
The response is PayUTransactionHistory
array list.
Check balance
Use the checkBalance
method to check registered account balance.
bolt.core.checkBalance(accountDetail: PayUAccountDetail, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail Parameters |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_BALANCE
Field | Definition |
---|---|
Balance | String Account balance |
Remove account
Use the removeAccount
method to remove registered account from your device.
bolt.core.removeAccount(accountDetail: PayUAccountDetail, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail Parameters |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_ACCOUNT_REMOVE_V3
Change mPIN
Use the changeMpin
method to change the mobile PIN of the registered account.
bolt.core.changeMpin(accountDetail: PayUAccountDetail, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
accountDetailmandatory | PayUAccountDetail Refer to PayU Account Detail Parameters |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_CHANGE_MPIN
Fetch VPA profile
Use the fetchVpaProfile
method to fetch the VPA profile.
bolt.core.fetchVpaProfile(vpa: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
vpamandatory | String VPA or UPI handle |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_PROFilE_VPA_V3
Save VPA
Use the saveVpa
method to save a VPA profile.
bolt.core.saveVpa(vpa: String, name: String, nickName: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
vpamandatory | String VPA or UPI handle |
namemandatory | String Name of customer |
nickNamemandatory | String Nickname for saving VPA |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_SAVE_VPA_V3
Delete VPA
Use the deleteVpa
method to delete a VPA profile.
bolt.core.deleteVpa(vpa: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
vpamandatory | String VPA or UPI handle |
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_VPA_REMOVE_V3
Raise query or dispute
Use the raiseQuery
method to raise query/ dispute for the transaction.
bolt.core.raiseQuery(txnId: String, txnRefId: String, amount: Double, query: String, callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Parameter | Description |
---|---|
txnIdmandatory | String Transaction ID and it should be unique for each transaction. |
txnRefIdmandatory | String Transaction Ref Id |
amountmandatory | String Total transaction amount. |
query mandatory | String Query or dispute description |
callback mandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_RAISE_QUERY_V3
Fetch query list
Use the fetchQueryList
method to fetch the query list.
bolt.core.fetchQueryList(callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_LIST_QUERIES_V3
Response Parameter | Definition |
---|---|
ArrayList<PayUTransactionHistory> | ArrayList List of PayU transaction history |
Deregister
Use the deregister
method to deregister your device from UPI.
bolt.core.deregister(callback: PayUUPIBoltCallBack)
The following fields are needed as a request for this API:
Fields | Definition |
---|---|
callbackmandatory | PayUUPIBoltCallBack Refer to Listener or Callback logic sub-section. |
Response: Response type is REQUEST_GET_CUSTOMER_DEREGISTER_V3
Check required permissions
Use the hasPermissions
method to check if all required permissions are granted.
Check clear cache
Use the clearCache
method to clear all ongoing callbacks.
Clear data
Use the clearData
method clears user data saved on device. It also clears the device binding.
PayU Account Detail Parameters
Parameter | Definition |
---|---|
nameoptional | Account Name |
accRefNumbermandatory | Account Reference Number |
ifscoptional | IFSC Code |
maskedAccnumberoptional | Masked Account Numner |
typeoptional | Account Type |
vpaoptional | - |
iinoptional | Account IIN |
mmidoptional | - |
aebaoptional | - |
mbebaoptional | - |
dLengthoptional | - |
dTypeoptional | - |
balanceoptional | - |
balTimeoptional | - |
statusoptional | - |
bankCodeoptional | Bank Code |
formatTypeoptional | - |
atmdLengthoptional | - |
bankNameoptional | Bank Name |
otpdTypeoptional | - |
otpdLengthoptional | - |
bankIdoptional | Bank ID |
Updated 21 days ago