The Eligible Bin for EMI API v2.0 is used only when the merchant needs the EMI feature of PayU. If you are managing card details on your website, this API can tell the issuing bank of the card bin. It also provides the minimum eligible amount for a particular bank.
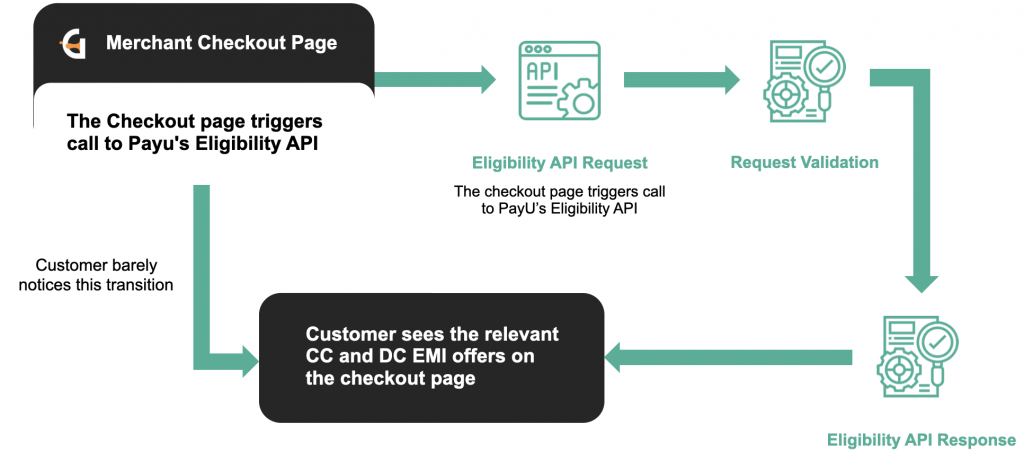
HTTP Method: POST
You can post a request using any of the following methods:
Request headers
The request header contains the following fields:
Field | Description | Example |
---|---|---|
Date mandatory | The date and time should be in the GMT time conversion(not the IST). For example, current time in India is 18:00:00 IST, the time in the date header should be 12:30:00 GMT. | Thu, 17 Feb 2022 08:17:59 GMT |
Digest | Base 64 encode of (sha256 hash of the JSON data (post to server). |
|
Authorization | This field is in the following format:
| hmac username="smsplus", algorithm="hmac-sha256", headers="date digest", signature="zGmP5Zeqm1pxNa+d68DWfQFXhxoqf3st353SkYvX8HI=" |
platformId | This field contains the platform ID and include the value as 1. | 1 |
The following sample Java code contains the logic used to encrypt as described in the above table:
import com.google.gson.Gson;
import com.google.gson.JsonObject;
import org.apache.commons.codec.binary.Base64;
import org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.security.InvalidKeyException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HmacAuth {
public static String getSha256(String input) {
try {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] digest = md.digest(input.getBytes());
return Base64.encodeBase64String(digest);
} catch (NoSuchAlgorithmException ignored) {}
return null;
}
public static JsonObject getRequestBody(){
JsonObject requestJson = new JsonObject();
requestJson.addProperty("firstname","John");
requestJson.addProperty("lastname","Doe");
return requestJson;
}
public static void main(String[] args) throws NoSuchAlgorithmException, InvalidKeyException {
String key = "smsplus";
String secret = "admin";
Gson gson = new Gson();
String date = DateTimeFormat.forPattern("EEE, dd MMM yyyy HH:mm:ss 'GMT'").withZoneUTC().print(new DateTime());
System.out.println(date);
JsonObject requestJson = getRequestBody();
String digest = getSha256(gson.toJson(requestJson));
System.out.println(digest);
String signingString = new StringBuilder()
.append("date: " + date)
.append("\ndigest: " + digest).toString();
Mac sha256_HMAC = Mac.getInstance("HmacSHA256");
SecretKeySpec secret_key = new SecretKeySpec(secret.getBytes(), "HmacSHA256");
sha256_HMAC.init(secret_key);
String signature = Base64.encodeBase64String(sha256_HMAC.doFinal(signingString.getBytes()));
String authorization = new StringBuilder()
.append("hmac username=\"")
.append(key)
.append("\", algorithm=\"hmac-sha256\", headers=\"date digest\", signature=\"")
.append(signature)
.append("\"").toString();
System.out.println(authorization);
}
}
Request without bank selection
Request parameters
Parameter | Description | Example |
---|---|---|
bintype mandatory | This parameter needs can include any of the following the values:
| bin |
value | This parameter can contain any of the following:
| 4161041969147181 |
amount | This parameter needs to include the transaction amount.
| 10000 |
Response parameters
Parameter | Description | Example |
---|---|---|
status | This parameter returns the status of web service call. | 1 |
msg | This parameter returns whether the EMI details were fetched successfully or not found. | Details fetched successfully |
details | The details of the EMI offer is displayed in a JSON format and it contains the following fields: |
|
Sample response
- If successfully fetched:
If successfully fetched:
{
"message": "Details fetched successfully",
"status": 1,
"result": [
{
"isEligible": 1,
"bank": "ICICI",
"minAmount": 1500.0
}
]
- If not found:
If not found:
{
"message": "Details fetched successfully",
"status": 1,
"result": [
{
"isEligible": 0,
"bank": "ICICI",
"minAmount": 1500.0
}
]
}
Request with bank selection
Request parameters
Parameter | Description | Example |
---|---|---|
bintype mandatory | This parameter needs can include any of the following the values:
| bin |
value | This parameter can contain any of the following:
| 4161041969147181 |
amount | This parameter needs to include the transaction amount. Note: Amount is a non-mandatory field, but is mandatory if bank code is ONEC or BAJFIN . | 10000 |
bank | This parameter contains the bank code for which the request is sent. | ICICI |
Response parameters
Parameter | Description | Example |
---|---|---|
status | This parameter returns the status of web service call. | 1 |
msg | This parameter returns whether the EMI details were fetched successfully or not found. | Details fetched successfully |
details | The details of the EMI offer is displayed in a JSON format and it contains the following fields:
| "isEligible": 0, |
Sample response
Success scenario
Formatted JSON Response:
If successfully fetched:
Array
(
[status] => 1
[msg] => Details fetched successfully
[details] => Array
(
[isEligible] => 1
[bank] => AXIS
[minAmount] => 2500
)
)
Failure scenarios
- If var3 (input bank name) does not match with the bank name in the PayU Database, the bin given in the input is of a different bank name:
Array (
[status] => 0
[msg] => Invalid Bin )
- When the BIN passed does not match with the bankName passed in the request:”
{
"message": "This Card does not belong to Axis Bank Credit Card. Please make the payment via ICICI Bank Credit Card",
"status": 0,
"result": [
{
"isEligible": 0,
"bank": "Axis"
}
]
}